Akkaアクターシステムの設定とSLF4Jによるアクターのログ取得
アクター間のメッセージの遣り取りを、SLF4Jによるログシステムにより確認します。スケジューラーにより5秒毎にメッセージの遣り取りが実行されます。
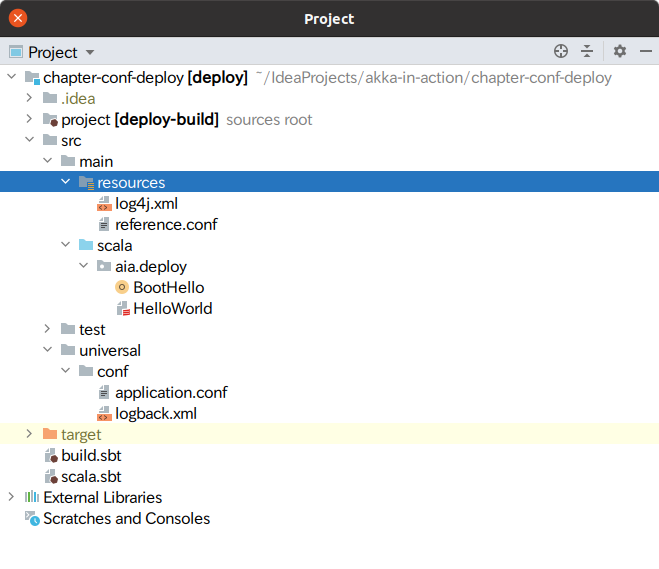
build.sbt
name := "deploy"
version := "1.0"
organization := "manning"
scalacOptions ++= Seq(
"-deprecation",
"-unchecked",
"-Xlint",
"-Ywarn-unused",
"-Ywarn-dead-code",
"-feature",
"-language:_"
)
enablePlugins(JavaAppPackaging)
scriptClasspath +="../conf"
libraryDependencies ++= {
val akkaVersion = "2.6.10"
Seq(
"com.typesafe.akka" %% "akka-actor" % akkaVersion,
"com.typesafe.akka" %% "akka-slf4j" % akkaVersion,
"ch.qos.logback" % "logback-classic" % "1.2.3",
"com.typesafe.akka" %% "akka-testkit" % akkaVersion % "test",
"org.scalatest" %% "scalatest" % "3.2.2" % "test"
)
}
scala.sbt
scalaVersion := "2.13.1"
scalacOptions ++= Seq(
"-deprecation",
"-unchecked",
"-Xfatal-warnings",
"-feature",
"-language:_"
)
appilication.conf
akka {
loggers = ["akka.event.slf4j.Slf4jLogger"]
# Options: ERROR, WARNING, INFO, DEBUG
loglevel = "DEBUG"
}
reference.conf
helloWorld {
timer=5000
}
BootHello.scala
package aia.deploy
import akka.actor.{ Props, ActorSystem }
import scala.concurrent.duration._
object BootHello extends App {
val system = ActorSystem("hello-kernel")
val actor = system.actorOf(Props[HelloWorld])
val config = system.settings.config
val timer = config.getInt("helloWorld.timer")
system.actorOf(Props(
new HelloWorldCaller(
timer = timer millis,
actor = actor)))
}
HelloWorld.scala
package aia.deploy
import akka.actor.{Actor, ActorLogging, ActorRef}
import scala.concurrent.ExecutionContextExecutor
import scala.concurrent.duration._
class HelloWorld extends Actor
with ActorLogging {
def receive: Receive = {
case msg: String =>
val hello = "Hello %s".format(msg)
sender() ! hello
log.info("Sent response {}",hello)
}
}
class HelloWorldCaller(timer: FiniteDuration, actor: ActorRef)
extends Actor with ActorLogging {
case class TimerTick(msg: String)
override def preStart(): Unit = {
super.preStart()
implicit val ec: ExecutionContextExecutor = context.dispatcher
context.system.scheduler.scheduleAtFixedRate(
timer,
timer,
self,
TimerTick("everybody"))
}
def receive: Receive = {
case msg: String => log.info("received {}",msg)
case tick: TimerTick => actor ! tick.msg
}
}
実行結果
[INFO] [11/30/2020 17:47:30.471] [hello-kernel-akka.actor.default-dispatcher-6] [akka://hello-kernel/user/$a] Sent response Hello everybody
[INFO] [11/30/2020 17:47:30.471] [hello-kernel-akka.actor.default-dispatcher-7] [akka://hello-kernel/user/$b] received Hello everybody
[INFO] [11/30/2020 17:47:35.439] [hello-kernel-akka.actor.default-dispatcher-5] [akka://hello-kernel/user/$a] Sent response Hello everybody
[INFO] [11/30/2020 17:47:35.439] [hello-kernel-akka.actor.default-dispatcher-7] [akka://hello-kernel/user/$b] received Hello everybody
[INFO] [11/30/2020 17:47:40.439] [hello-kernel-akka.actor.default-dispatcher-5] [akka://hello-kernel/user/$a] Sent response Hello everybody
[INFO] [11/30/2020 17:47:40.439] [hello-kernel-akka.actor.default-dispatcher-7] [akka://hello-kernel/user/$b] received Hello everybody
[INFO] [11/30/2020 17:47:45.438] [hello-kernel-akka.actor.default-dispatcher-7] [akka://hello-kernel/user/$a] Sent response Hello everybody
[INFO] [11/30/2020 17:47:45.438] [hello-kernel-akka.actor.default-dispatcher-5] [akka://hello-kernel/user/$b] received Hello everybody
........................
........................